Breaking Down Binary Search: A Beginner's Guide
December 20, 2023 • 0 minute read •
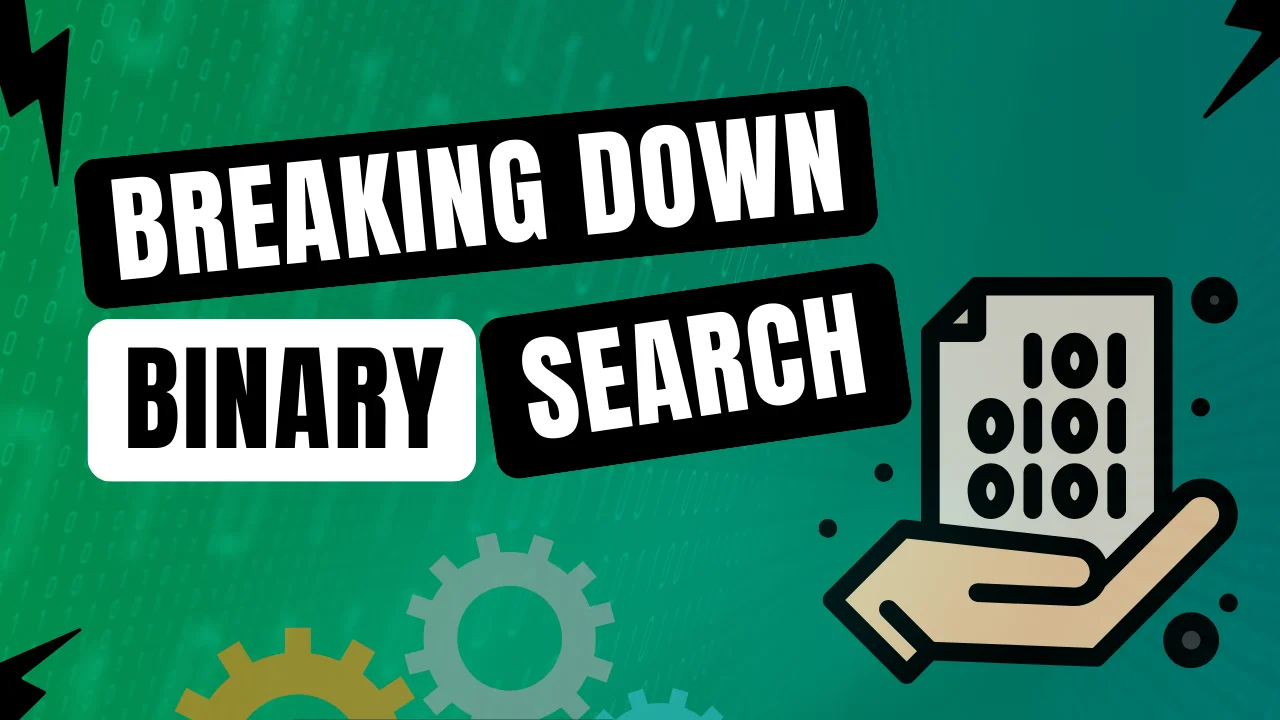
Binary search is a fundamental algorithm in computer science used for efficiently finding a specific item in a sorted list of items. It works by repeatedly dividing in half the portion of the list that could contain the item until the possible locations have been reduced to just one.
In programming, binary search is crucial due to its efficiency. Compared to linear search, which scans each item in a list one by one, binary search reduces the time it takes to find an item significantly, especially in large lists. This efficiency makes it a go-to method for searching in sorted arrays and is a basic concept that underpins more complex algorithms and data structures.
In this article, we'll discuss the concept of binary search in detail. We'll explain how it works, its efficiency, and how to implement it in Python. Let's get started!
What is Binary Search?
Binary search is a technique used to find a specific element in a sorted list. It does this by repeatedly dividing the range of possible locations in half until it locates the item or determines that the item isn't in the list.
A key point about binary search is that it only works with sorted lists. The list must be ordered in some way (numerically, alphabetically, etc.) for binary search to function correctly.
To illustrate, imagine searching for a word in a dictionary. Instead of going through each word from the start, you open the dictionary to the middle. If the word you're looking for should come before this middle point (alphabetically), you then focus on the first half of the dictionary. If it should come after, you focus on the second half. You keep halving the relevant section like this until you find the word or conclude that it's not in the dictionary. Binary search applies this halving strategy to quickly locate items in any sorted list.
Binary Search Steps
The binary search algorithm can be broken down into the following steps:
a. Understand the Problem: Ensure the list is sorted. Binary search won't work correctly on unsorted data.
b. Set Up Pointers: Initialize two pointers, usually named left
and right
. left
starts at the beginning of the list (index 0), and right
starts at the end (index length - 1).
c. Start Searching:
- Calculate the middle index:
middle = (left + right) / 2
. (In programming, ensure this is integer division.) - Compare the element at the
middle
index with the target value. - If the middle element is the target, you've found the item.
- If the middle element is greater than the target, move the
right
pointer tomiddle - 1
. - If the middle element is less than the target, move the
left
pointer tomiddle + 1
.
d. Repeat Until Found or Pointers Cross: Continue this process of dividing and comparing. If left
exceeds right
, the target is not in the list.
e. Wrap It Up: Return the result. This could be the index of the found element or an indication that the element is not in the list.
Programming Example:
Here's a Python example of a binary search function with explanations in comments:
def binary_search(arr, target):
left, right = 0, len(arr) - 1 # Initialize pointers
while left <= right:
middle = (left + right) // 2 # Calculate middle index
# Check if the middle element is the target
if arr[middle] == target:
return middle # Target found, return its index
# Decide which half to continue searching in
if arr[middle] < target:
left = middle + 1 # Search in the right half
else:
right = middle - 1 # Search in the left half
return -1 # Target not found
# Example usage
arr = [1, 3, 5, 7, 9]
target = 5
print(binary_search(arr, target)) # Outputs: 2 (index of target)
In this example, the function binary_search
takes a sorted array arr
and a target
value. It uses binary search to find the index of the target
in arr
, returning the index if found, or -1 if not found. Notice that when calculating the middle
index, we use integer division to ensure we get an integer result. This is important because we use the middle
index to access the array elements.
Efficiency of Binary Search
Binary search is highly efficient for several reasons:
-
Reduced Search Space: Unlike linear search, which goes through each element one by one, binary search eliminates half of the remaining elements in each step. This drastically reduces the number of comparisons and searches needed.
-
Time Complexity: The time complexity of binary search is O(log₂ N), where N is the number of elements in the list. This notation means that the time taken to search increases logarithmically with the size of the list. In practical terms, even if the list size doubles, it only requires one extra comparison step.
-
Comparison with Linear Search: In contrast, linear search has a time complexity of O(N), meaning the time taken increases linearly with the list size. For small datasets, this difference might not be noticeable, but as the dataset grows, the efficiency of binary search becomes significantly better. For example, for a list of 1,000,000 elements, a linear search could potentially make 1,000,000 comparisons in the worst case, whereas binary search would require at most around 20 comparisons (since log₂(1,000,000) is approximately 20).
In summary, the logarithmic time complexity of binary search makes it an extremely efficient algorithm for searching through large, sorted datasets, vastly outperforming linear search as the size of the dataset increases.
Handling Duplicates in Binary Search
When binary search encounters duplicate elements, it does not inherently guarantee finding the first occurrence of the element. By its nature, binary search targets finding any occurrence of the element, which might not be the first one if duplicates are present.
If the specific requirement is to find the first occurrence of a duplicate element, the binary search needs to be modified slightly:
- Find Any Occurrence: First, use binary search to find any occurrence of the target element.
- Find the First Occurrence: Once an occurrence is found, continue searching in the left half (i.e., elements before the found occurrence) to check if there are earlier occurrences.
Here's a Python function that implements this approach:
def binary_search_first_occurrence(arr, target):
left, right = 0, len(arr) - 1
result = -1 # Default if target is not found
while left <= right:
middle = (left + right) // 2
if arr[middle] == target:
result = middle # Found an occurrence
right = middle - 1 # Continue searching to the left
elif arr[middle] < target:
left = middle + 1
else:
right = middle - 1
return result # Either the first occurrence index or -1
# Example usage
arr = [1, 2, 4, 4, 4, 5, 6]
target = 4
print(binary_search_first_occurrence(arr, target)) # Outputs: 2
In this code, when an occurrence of the target is found, instead of immediately returning, the algorithm narrows the search to the left portion of the array. This ensures that if an earlier occurrence of the target exists, it will be found, effectively locating the first occurrence in the case of duplicates.
Recursive Binary Search
Recursive binary search is a variation of the traditional binary search algorithm that utilizes recursion instead of iteration. In recursion, the function calls itself with a smaller subset of the data until it finds the target or concludes it's not present.
Function and Parameters:
The recursive binary search function typically has the following parameters:
arr
: The sorted array in which we are searching.target
: The element we are searching for.left
: The starting index of the array segment being searched.right
: The ending index of the array segment being searched.
Base Case:
The base case is crucial in recursive functions to prevent infinite recursion. For binary search, the base case occurs when the left
index exceeds the right
index, indicating the element isn't in the array.
Recursive Steps:
- Calculate Middle: Find the middle index of the current array segment.
- Compare: Check if the middle element is the target.
- If it is, return its index.
- Recursion:
- If the middle element is greater than the target, recursively search the left half.
- If it is less, recursively search the right half.
Python Example:
Here's a Python implementation of recursive binary search with explanations:
def recursive_binary_search(arr, target, left, right):
if left > right:
return -1 # Base case: target not found
middle = (left + right) // 2
if arr[middle] == target:
return middle # Target found
elif arr[middle] < target:
return recursive_binary_search(arr, target, middle + 1, right) # Search right half
else:
return recursive_binary_search(arr, target, left, middle - 1) # Search left half
# Example usage
arr = [1, 3, 5, 7, 9]
target = 5
print(recursive_binary_search(arr, target, 0, len(arr) - 1)) # Outputs: 2
In this example, the recursive_binary_search
function is called initially with the full range of the array (left
as 0 and right
as len(arr) - 1
). Depending on the comparison with the middle element, it calls itself with either the left or the right half of the array, continually narrowing down the search area until the target is found or the base case is reached.
Practical Application of Binary Search
Binary search is a versatile and efficient algorithm with numerous real-world applications, particularly in areas where quick search operations are crucial. Here are some of its practical uses:
-
Searching in Databases:
- In database systems, binary search is often employed to quickly locate records in sorted datasets. For instance, when a database index is sorted, binary search can rapidly find specific records within that index, significantly speeding up query response times.
- It's especially useful in large databases where linear search would be too slow. By using binary search, the time taken to find a record scales logarithmically with the size of the dataset, making it ideal for databases with millions or even billions of records.
-
Implementing Autocomplete in Search Engines:
- Search engines and various software applications use binary search to implement autocomplete features. When a user starts typing in a search box, the system needs to quickly find matching suggestions from a large, sorted list of keywords or phrases.
- By using binary search, the application can efficiently narrow down the potential suggestions, offering real-time feedback to the user. This not only improves user experience but also optimizes the computational resources needed for such a feature.
-
Finding Elements in Sorted Arrays or Lists:
- In software development, binary search is commonly used to find elements in sorted arrays or lists. This is applicable in numerous scenarios, such as searching for a specific user in a sorted list of users, finding a book by its title in a sorted library catalog, or locating a particular data point in a sorted array of sensor data.
- Its efficiency in dealing with sorted data structures makes it a preferred method in situations where data is frequently searched and performance is a key concern.
These are just a few examples of how binary search is used in real-world applications. Its efficiency and versatility make it a fundamental algorithm in computer science and programming.
Conclusion
In this article, we've explored the binary search algorithm in depth, covering its fundamental concepts, steps, and practical applications. Key points include:
-
Binary Search Basics: We introduced binary search as an efficient method for finding elements in sorted lists, emphasizing its reliance on a sorted dataset.
-
Steps and Efficiency: The step-by-step process of binary search, including setting up pointers, calculating the middle, and updating pointers based on comparisons, was outlined. We highlighted its efficiency with a time complexity of O(log₂ N), making it significantly faster than linear search for large datasets.
-
Handling Duplicates: We discussed how binary search can be adjusted to handle duplicates, specifically focusing on finding the first occurrence of an element.
-
Recursive Approach: The recursive version of binary search was explained, showing how the algorithm can be implemented using self-calls to narrow down the search space.
-
Practical Applications: Real-world applications of binary search in databases, autocomplete features, and finding elements in sorted data structures were discussed, demonstrating its versatility and utility in various fields.
In conclusion, binary search stands out for its efficiency and practicality, especially in handling large sorted datasets. It's a fundamental algorithm that's worth mastering for any programmer.
If you liked this article, hit the like button and let me know in the comments below. I'd love to hear your thoughts!